OpenSCAD tasks
Solutions of both OpenSCAD lessons tasks - you can get extra point for non-trivial Pull Request correcting a mistake or making things easier.
Easy ones
You should be fine with:
- 3D primitives (cube, cylinder, sphere, no polyhedron)
- CSG modeling (union, difference, intersection)
- transformations (rotate, translate, scale, resize, mirror, no multimatrix, hull or minkowski)
- for (and intersection_for), if (ternary operator), variables
- modules
- math operators and function
STLs: stls.zip
/** * Clip * clip for pouch securing * @param x total length of the clip * @param y total width of the clip * @param z total height of the clip * @param tooth one tooth side size * @param off offset from the closed side * @param distance distance between teeth */ module clip (x=60,y=20,z=5,tooth=9,off=6,distance=8) {} /** * Dice * Basic shape is intersection of a cube and sphere * Use for loops to create dots * @param cs Cube size (side) * @param ds Dot size (radius) */ module dice(cs=40, ds=3) {} /** * Parametric drill stand * Use for loops * @param base_height Base height * @param holes Number of holes (each one is 1 mm wider) * @param hole_depth Depth of the holes * @param stand_step_width One line of holes width * @param stand_step_num Holes per line */ module drill_stand( base_height=10, holes=10, hole_depth=5, stand_step_width=15, stand_step_num=5 ) {} /** * Parametric gear * Use for loop for teeth! * @param gear_rad Gear radius * @param gear_thickness Gear thickness * @param center_hole_width Middle square size * @param tooth_width Tooth width * @param tooth_prot Tooth overlap (how much it goes out of the wheel) * @param tooth_count Number of teeth */ module gear( gear_rad=50, gear_thickness=10, center_hole_width=10, tooth_width=5, tooth_prot=5, tooth_count=20 ) {} /** * Hypercube * @author Tomáš Kasalický * @param a1 Outer size of outer cube * @param a2 Outer size of inner cube * @param th Rod with (side of the square profile) */ module hypercube(a1=20,a2=10,th=1) {} /** * Chair * Simple chair with rungs in backrest. * Use for loops! * @param rung_number Rung count * @param seat_width Width (and length) of the seat * @param seat_thickness Seat thickness * @param feet_width Feet and rungs thickness * @param feet_length Feet length. Starting form bottom part of the seat! */ module chair( rung_number=5, seat_width=19, seat_thickness=2, feet_width=2, feet_length=15 ) {} /** * Lego brick * @documentation http://cdn.instructables.com/F65/PI3W/HDYZBK5Y/F65PI3WHDYZBK5Y.LARGE.jpg * @param num_x Number of pins along X axis * @param num_y Number of pins along Y axis * @param num_z Height of the brick. In LEGO units. Common piece has 3 units. * @param smooth Should the brick be smooth (i.e. no pins) * * Bottom side might be hollow. */ module lego_brick(num_x=10,num_y=2,num_z=1,smooth=false) {} /** * Mug * @param r_mug Inner radius of the mug * @param z Mug height * @param z_base Base height * @param r_handle Inner radius of the handle * @param w_handle Handle width * @param thick Wall thickness */ module mug (r_mug=12,z=30,z_base=3,r_handle=10,w_handle=4,thick=1.5) {} /** * Parametric pen holder * Create holes by for loops! * @param bottom_rad Bottom radius * @param base_height Base height * @param top_rad Top radius * @param hole_count Hoel count * @param hole_rad Hole radius * @param prot_thickness Hole wall thickness * @param prot_angle Angle of holes */ module pen_holder( bottom_rad=50, base_height=50, top_rad=25, hole_count=5, hole_rad=10, prot_thickness=2, prot_angle=20 ) {} /** * Plate * Rectangular plate for electronic device with corner screw holes * @param x Plate width * @param y Plate length * @param z Plate height * @param c Hole center distance form sides * @param r Hole radius * @param b Pillar radius * @param h Pillar height */ module plate(x=70,y=90,z=2,c=5,r=1.5,b=2.5,h=3) {} /** * Parametric SIM card adapter * @param x1,y1 outer adapter dimensions * @param x2,y2 inner adapter dimensions * @param z adapter height * @param off_x,off_y offset of the hole * Don't forget the corner * If the hole doesn't fit in, make the outer part large enough * The corner cut line should come trough 3/4 of Y side. */ module sim_card(x1=18,y1=14,x2=10,y2=8,z=1,off_x=1,off_y=1) {} /** * Snowman * @param r Biggest snowball radius * @param factor Size of a smaller snowball as a fraction of the bigger one (0.7 = 70 %) * @param overlap Snowballs height overlap as a fraction of height of the bigger snowball (0.2 = 20 %) * Only make 3 snowballs on top of each other, no decorations necessary */ module snowman(r=50,factor=0.7,overlap=0.2) {} /** * Parametric wall hook * Create holes by for loops! * @param hook_rad Hook curve INNER radius * @param hook_thickness Hook thickness (depth from wall) * @param hook_width Hook width * @param top_hook_angle Angle of top part (for coats) * @param top_hook_length Length of top part (for coats) * @param top_hook_distance Flat part height (distance form top part to curve) * @param hole_rad Mount holes radius * @param hole_count Number of mount holes */ module wall_hook( hook_rad=10, hook_thickness=10, hook_width=10, top_hook_angle=30, top_hook_length=50, top_hook_distance=30, hole_rad=2, hole_count=2 ) {}
Harder ones
Arm
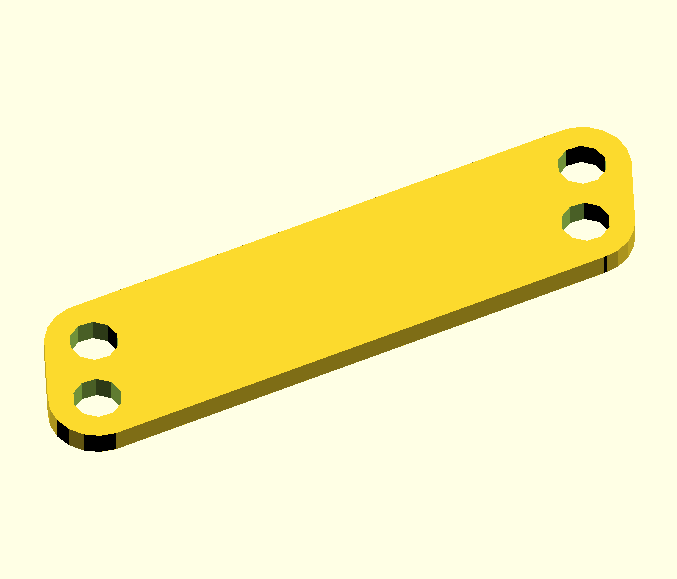
/**
* Mechanical arm
* @param h Arm height (counted from holes centers)
* @param off_set Top and bottom part offset
* @param thick Arm thickness
* @param number_holes Number of holes on each side
* @param hole_radius Holes radius and distance (from each other and from border)
* @author Jakub Průša
*/
module arm(
height=60,
offset=-25,
thick=3,
number_holes=2,
hole_radius=3
) {
//insert your code here...
}
Batman
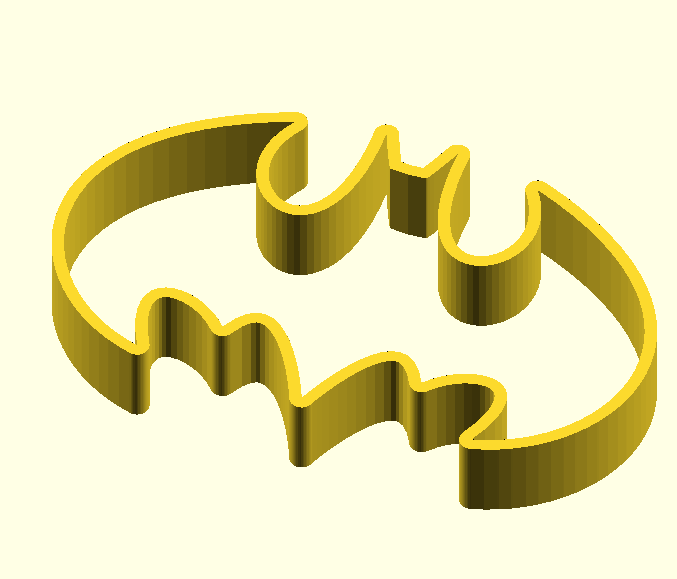
/**
* Batman cookie cutter
* @param x/y/z Outer size along X/Y/Z axis
* @param thick Wall thick
* @author Jakub Průša, Miro Hroncok
*/
module batman(
x=100,
y=60,
z=15,
thick=2
) {
//insert your code here...
}
Disc
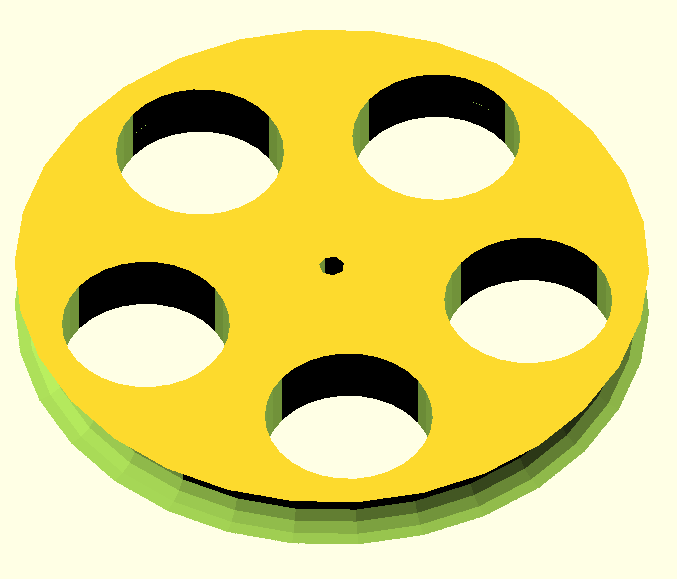
/**
* Parametric robot wheel
* @param radius Disc radius
* @param height Disc height
* @param r_hole Central hole radius
* @param reduce_offset Reduce holes offset from border
* @param reduce_num Number of reduce holes
* Reduce hole size? Make them fit.
* @author Marek Žehra
*/
module wheel (
radius=50,
height=10,
r_hole=2,
reduce_offset=5,
reduce_num=4
) {
//insert your code here...
}
Helix
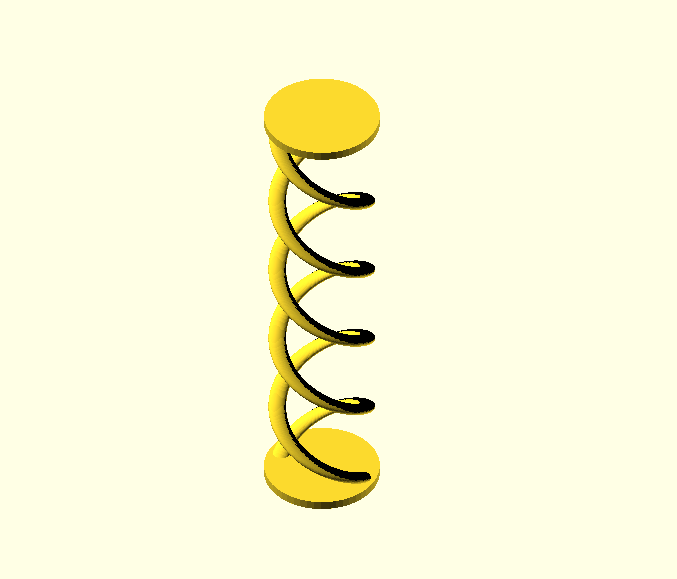
/**
* Helix
* @param d Helix distance from each other (center to center, measured on XY plane)
* @param o Helix radius (measured on XY plane)
* @param h Helix height without base
* @param s Rotation in degrees on one mm of height (positive or negative direction)
* @param db Base diameter
* @param hb Base height
* One slice should have 50 µm
* @author Miro Hrončok
*/
module helix(d=10,o=1,h=50,s=18,db=13,hb=1) {
//insert your code here...
}
Snowman
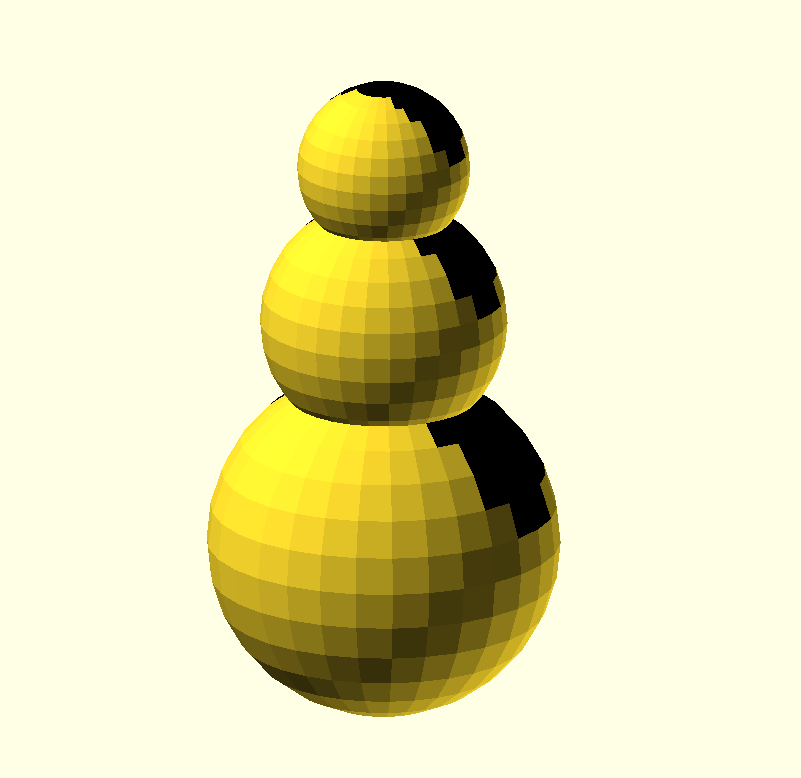
/**
* Snowman
* @param r Biggest snowball radius
* @param factor Size of a smaller snowball as a fraction of the bigger one (0.7 = 70 %)
* @param overlap Snowballs height overlap as a fraction of height of the bigger snowball (0.2 = 20 %)
* @param balls Number of snowballs (use recursion)
* Only make snowballs on top of each other, no decorations necessary
* @author Miro Hrončok
*/
module snowman(r=50,factor=0.7,overlap=0.2,balls=3) {
//insert your code here...
}