Basic Tasks for OpenSCAD tutorial
Clasification
- Complete solution (1 point)
- Submission doesn’t comply with task (0 points)
Possible point loss
- Task has to be written inside its own module (jinak 0 bodů)
- Task doen’t work when changing parameter (-0,5/(number of parameters) point)
Úlohy
Wall hook
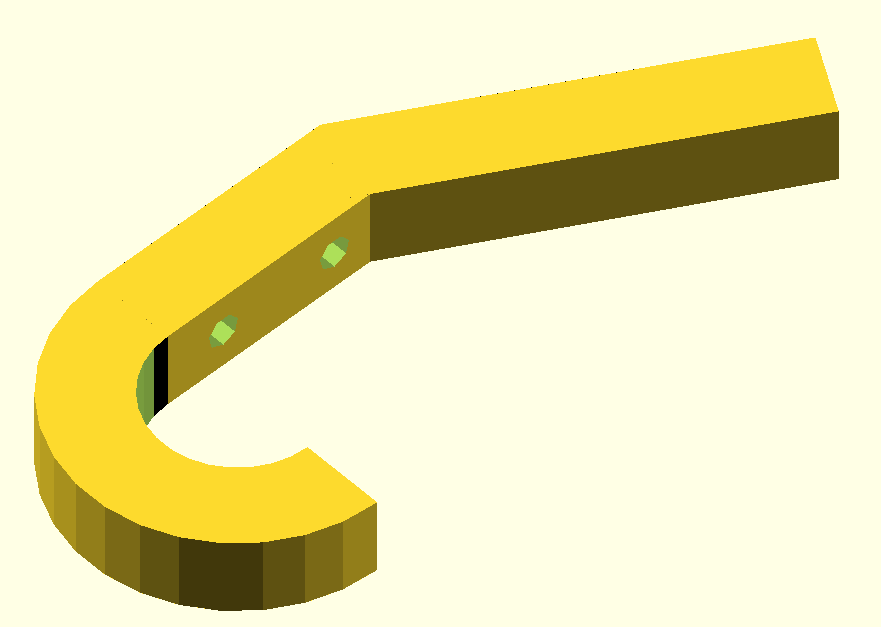
/**
* Parametric wall hook
* háčku na stěnu s možností modifikace parametrů
* Holes thought hook has to be generated using for loop
* @param hook_rad INNER radius of the hook
* @param hook_thickness Thickness of hook (measured from the wall)
* @param hook_width Hook Width
* @param top_hook_angle Angle of the top part of hook (the thing you hang hats on)
* @param top_hook_length Length of top part
* @param top_hook_distance Distance bottom and top part (Length of straight part with holes)
* @param hole_rad Radius of holes for screws
* @param hole_count Amount of holes for screws
* @author Marek Žehra
*/
module wall_hook(
hook_rad=10,
hook_thickness=10,
hook_width=10,
top_hook_angle=30,
top_hook_length=50,
top_hook_distance=30,
hole_rad=2,
hole_count=2
) {
//insert your code here...
}
Snowman

/**
* Snowman
* @param r Radius of the biggest sphere
* @param factor Size of smaller sphere is a factor of size the sphere above (0.7 = 70 %)
* @param overlap Overlap od smaller sphere with the sphere under as factor lower sphere (0.2 = 20 %)
* @author Miro Hrončok
*/
module snowman(r=50,factor=0.7,overlap=0.2) {
//insert your code here...
}
Simcard adapter

/**
* Parametric Adapter for Sim card
* @author Jakub Prusa
* @param x1,y1 outter sizes of adapter
* @param x2,y2 outter sizes of sim card
* @param z height of adapter
* @param off_x,off_y is offset from bottom corner
* Cutted corner shall be intersecting y size in its 3/4
*/
module sim_card(x1=18,y1=14,x2=10,y2=8,z=1,off_x=1,off_y=1) {
//insert your code here...
}
Plate

/**
* Plate
* Electronics base with 4 screw holes
* @param x width of plate
* @param y length of plate
* @param z thickness of plate
* @param c distance of screw from corner
* @param r radius of screw hole
* @param b radius of screw pillar
* @param h height of screw pilla
* @author Miro Hrončok, Jakub Průša
*/
module plate(x=70,y=90,z=2,c=5,r=1.5,b=2.5,h=3) {
//insert your code here...
}
Pen holder
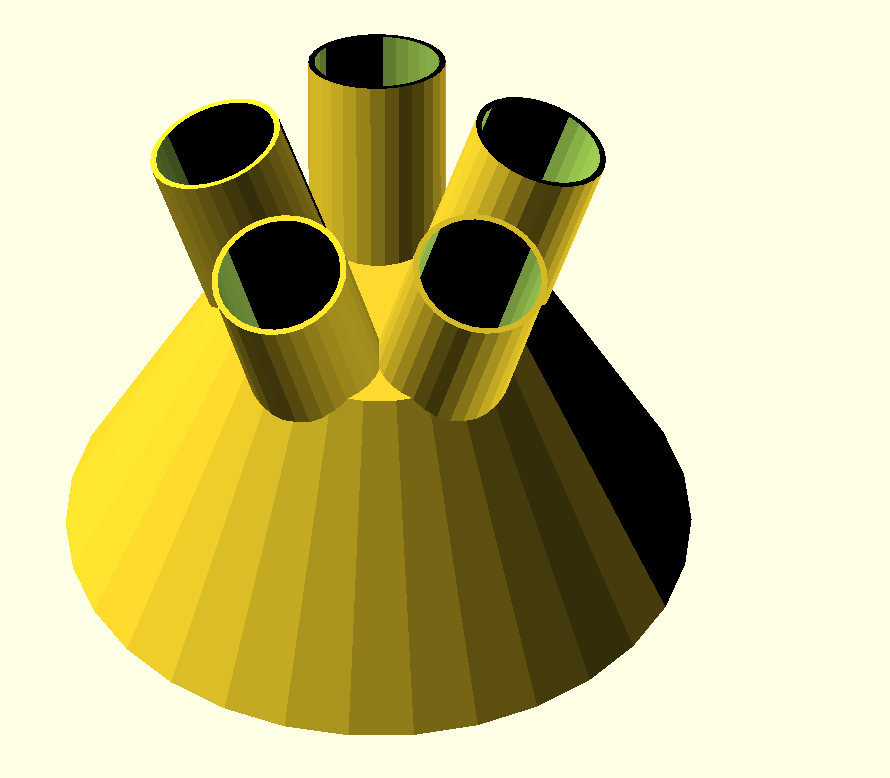
/**
* Parametric pen holder
* Holes needs to be done using for loop
* @param bottom_rad radius of base
* @param base_height base height
* @param top_rad top base radius
* @param hole_count Count of holes for pens
* @param hole_rad radius of pen holes
* @param prot_thickness thickness of pen holes
* @param prot_angle angle of pen holes
* @author Marek Žehra
*/
module pen_holder(
bottom_rad=50,
base_height=50,
top_rad=25,
hole_count=5,
hole_rad=10,
prot_thickness=2,
prot_angle=20
) {
//insert your code here...
}
Mug
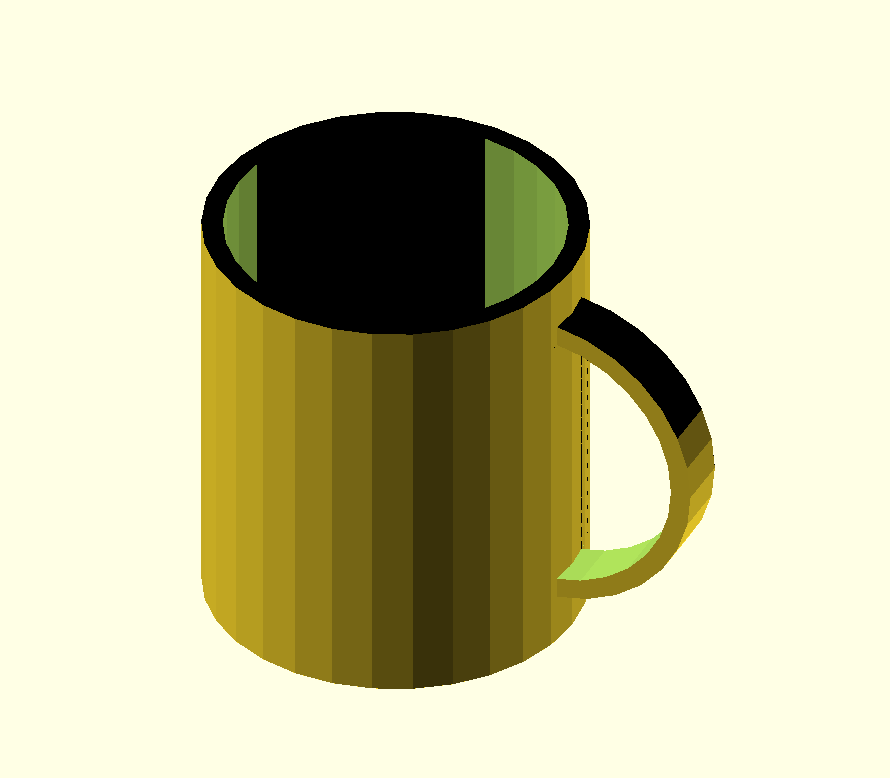
/**
* Mug
* @param r_mug INNER radius
* @param z height
* @param z_base thickness of base
* @param r_holder inner radius of holder
* @param w_holder thickness of holder
* @param thick mug wall thickness
* @author Jakub Průša
*/
module mug (r_mug=12,z=30,z_base=3,r_holder=10,w_holder=4,thick=1.5) {
//insert your code here...
}
Lego brick

/**
* Lego brick
* @author Jakub Prusa
* @documentation http://cdn.instructables.com/F65/PI3W/HDYZBK5Y/F65PI3WHDYZBK5Y.LARGE.jpg
* @param num_x number of pin on X axis
* @param num_y number of pin on Y axis
* @param num_z height on Z axis but not in mm, it should be in LEGO units one basic lego brick has height of 3
* @param smooth smooth means there will not be any pins
* dimenstions
* diameter of pin is 4.8 mm
* height of pin is 1.8 mm
* distance of pins is 8 mm
* height of layer without pins is 3.2 mm
* wall thickness is 1.2 mm
*
* There doenst have to be any pins inside the model.
*/
module lego_brick(num_x=10,num_y=2,num_z=1,smooth=false) {
//insert your code here...
}
Chair

/**
* Chair
* Back rest needs to be done using for loops
* @param rung_number Number of rungs
* @param seat_width width and length of the part that is touching your butt
* @param seat_thickness thickness of seat
* @param feet_width Thickness of feet and rungs
* @param feet_length length of legs, counter from bottom of seat
* @author Marek Žehra
*/
module chair(
rung_number=5,
seat_width=19,
seat_thickness=2,
feet_width=2,
feet_length=15
) {
//insert your code here...
}
4D Hypercube
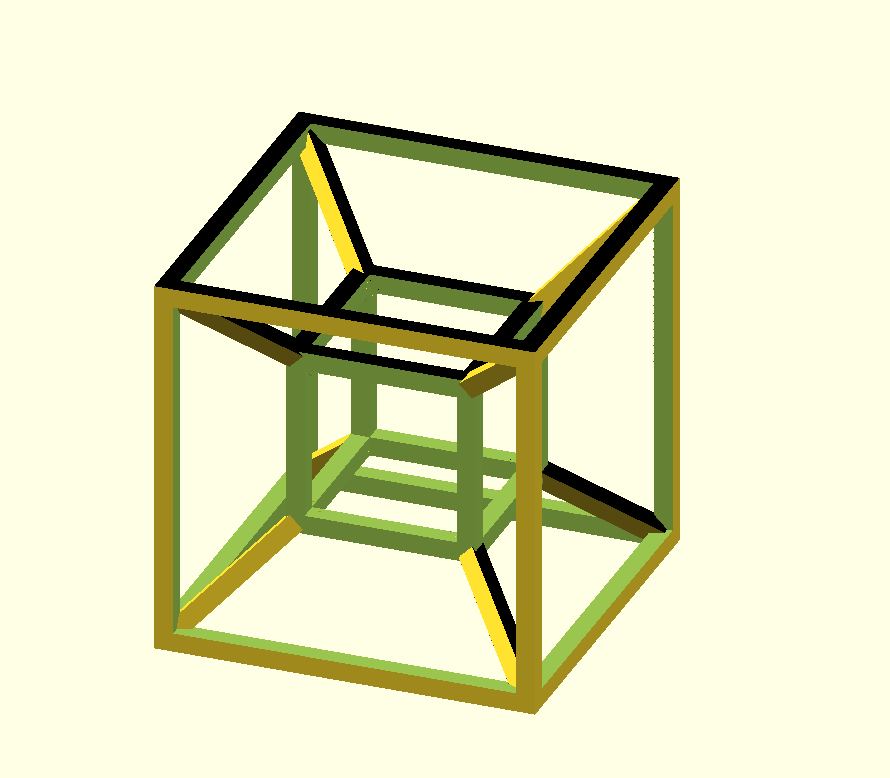
/**
* Hypercube
* @author Tomáš Kasalický
* @param a1 OUTTER size of OUTTER cube
* @param a2 OUTTER size of INNER cube
* @param th thickness
*/
module hypercube(a1=20,a2=10,th=1) {
//insert your code here...
}
Gear
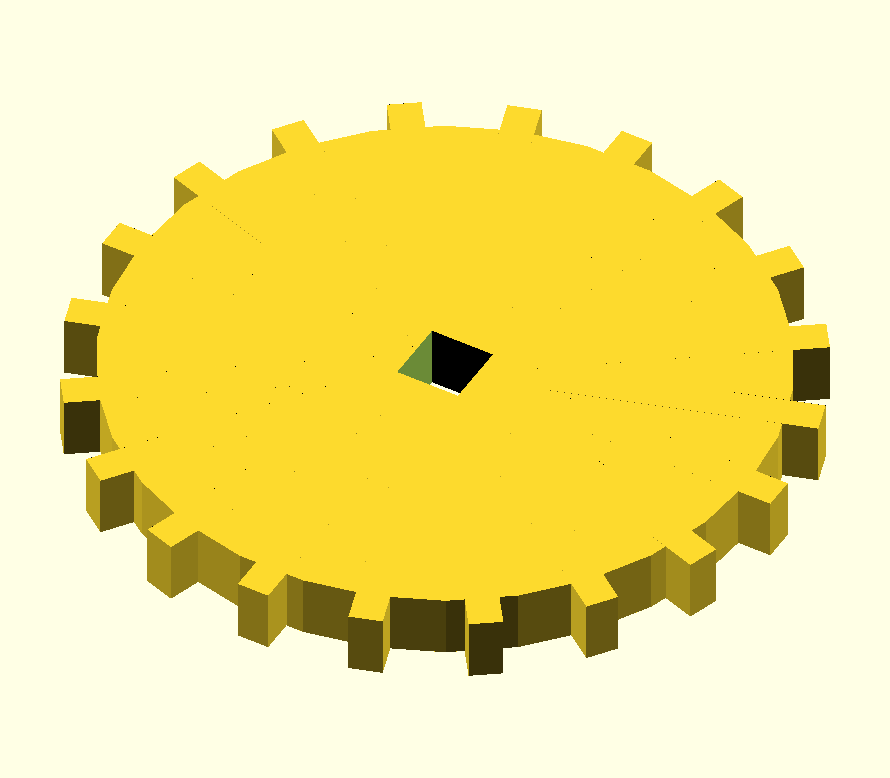
/**
* Parametric gear
* Teeths needs to be done using for loop
* @param gear_rad radius of gear
* @param gear_thickness thickness of gear
* @param center_hole_width width of center hole
* @param tooth_width width of tooth
* @param tooth_prot Tooth offset form the gear
* @param tooth_count Number of teeth on gear
* @author Marek Žehra
*/
module gear(
gear_rad=50,
gear_thickness=10,
center_hole_width=10,
tooth_width=5,
tooth_prot=5,
tooth_count=20
) {
//insert your code here...
}
Drill stand

/**
* Parametric drill stand
* Needs to be done using for loop
* @param base_height height of base
* @param holes count of holes
* @param hole_length length of holes
* @param stand_step_width width of one row
* @param stand_step_num amount of holes per row
* @author Marek Žehra
*/
module drill_stand(
base_height=10,
holes=10,
hole_length=5,
stand_step_width=15,
stand_step_num=5
) {
//insert your code here...
}
Dice
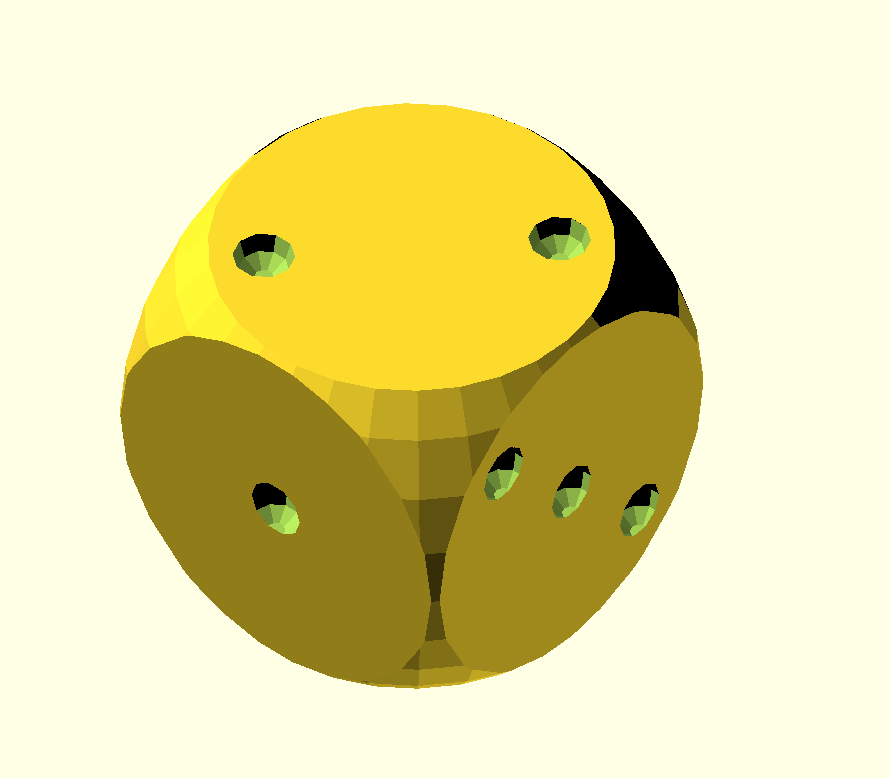
/**
* Dice
* Dots needs to be done using for loop
* @param cs cube size
* @param ds diameter of dot
* @author Tomáš Bařtipán, Miro Hrončok
*/
module dice(cs=40, ds=3) {
//insert your code here...
}
Clip
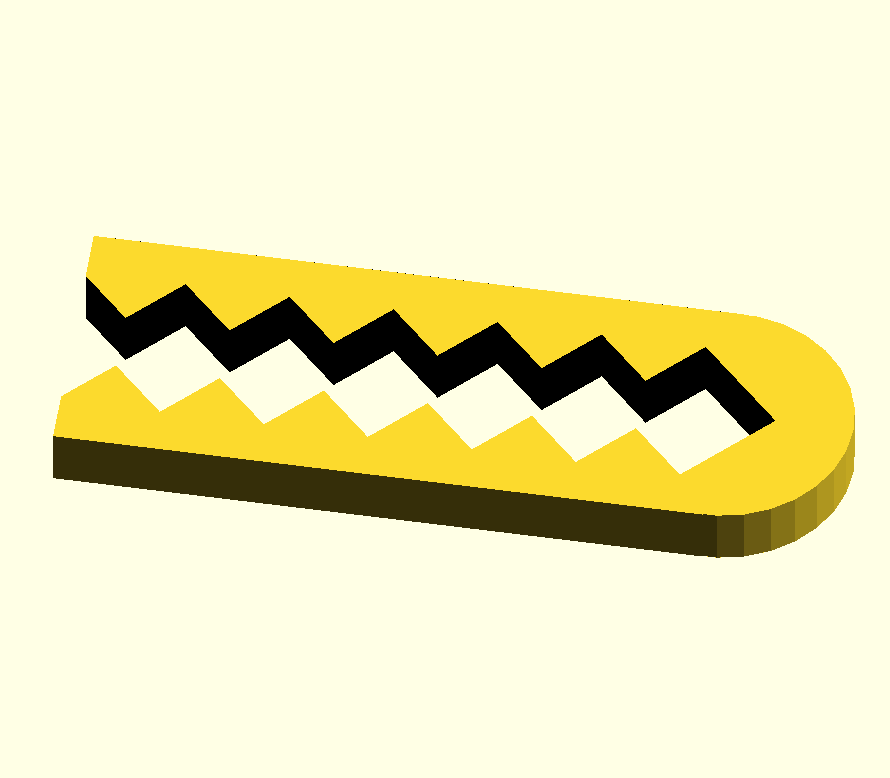
/**
* Clip
* @param x length of clip
* @param y width of clip
* @param z height of clip
* @param tooth side of one teeth
* @param off offset from right where the cylinder is
* @param distance between teeth
* @author Jakub Průša
*/
module clip (x=60,y=20,z=5,tooth=9,off=6,distance=8) {
//insert your code here...
}